Getting Started
Core Concepts
Reference
Troubleshooting
Feedback
PHP + Laravel - Starter Template
Deploy a starter template PHP app using the Laravel framework on Aptible
Overview
This guide will walk you through the process of launching a PHP app using the Laravel framework.
Quick Links
Deploy Template
⚠️ Prerequisite: Ensure you have Git installed.
Using the Deploy Code tool in the Aptible Dashboard, you can deploy the Laravel Template. The tool will guide you through the following:
Step 01: Deploy with Git Push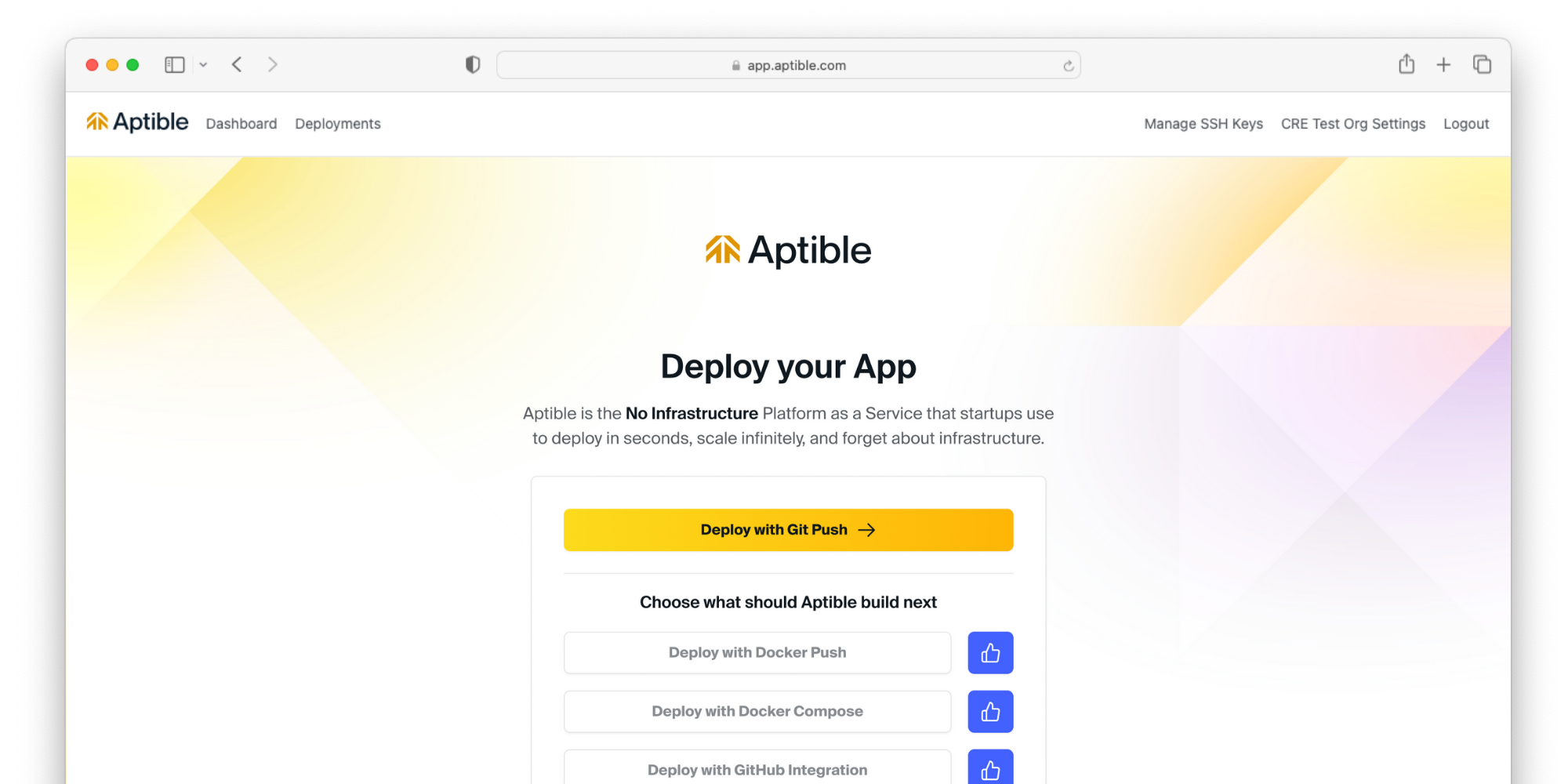
Step 02: Add an SSH key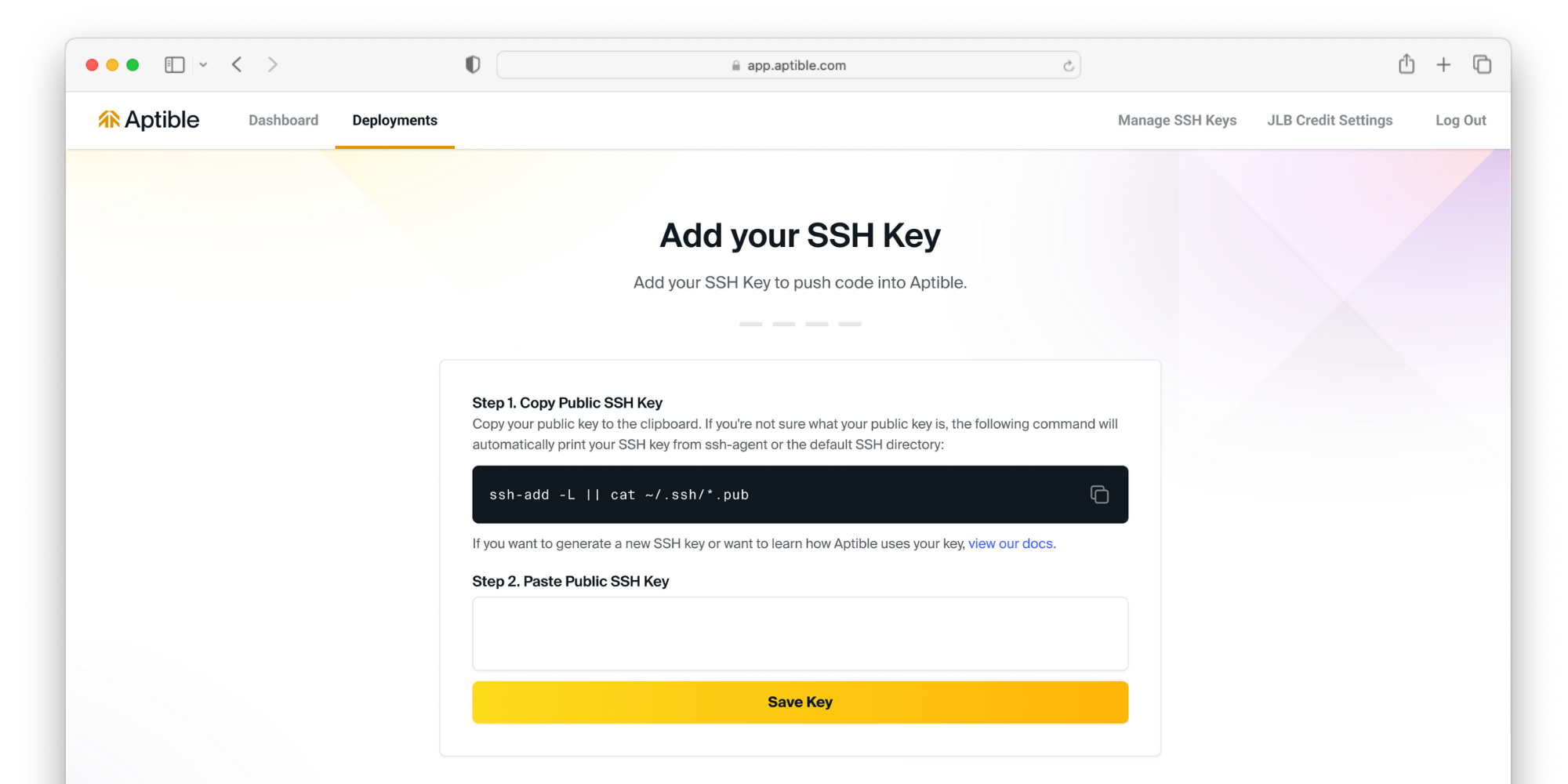
If you have not done so already, you will be prompted to set up an SSH key.
Step 03: Environment Setup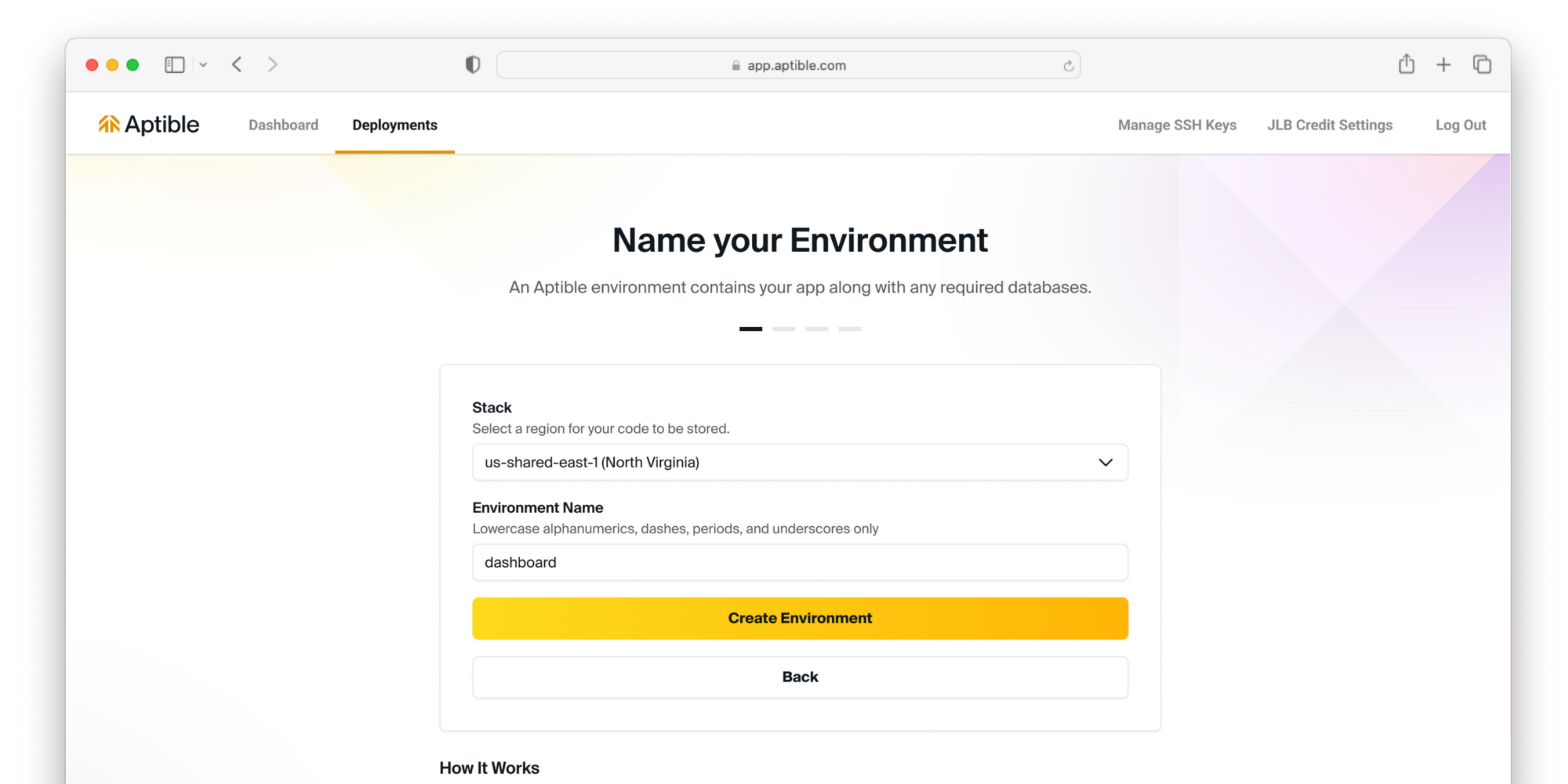
Select your stack to deploy your resources. This will determine what region your resources are deployed to. Then, name the environment your resources will be grouped into.
Step 04: Prepare the template
Select Laravel Template for deployment, and follow command-line instructions.
Step 05: Fill environment variables and deploy!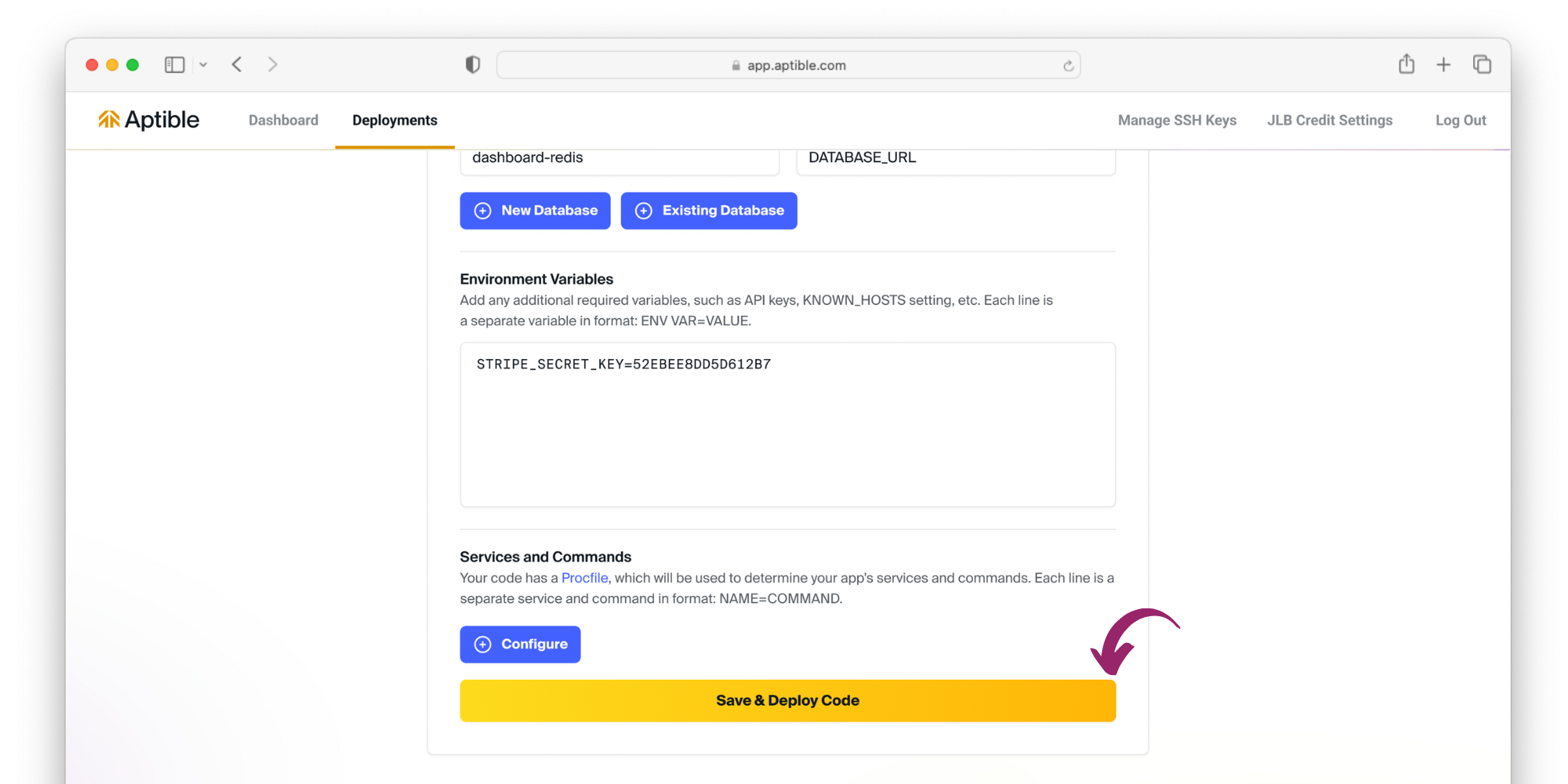
Aptible will automatically fill this template's required databases, services, and app's configuration with environment variable keys for you to fill with values. Once complete, save and deploy the code!
Step 06: View logs in real time.png)
Step 07: Expose your app to the internet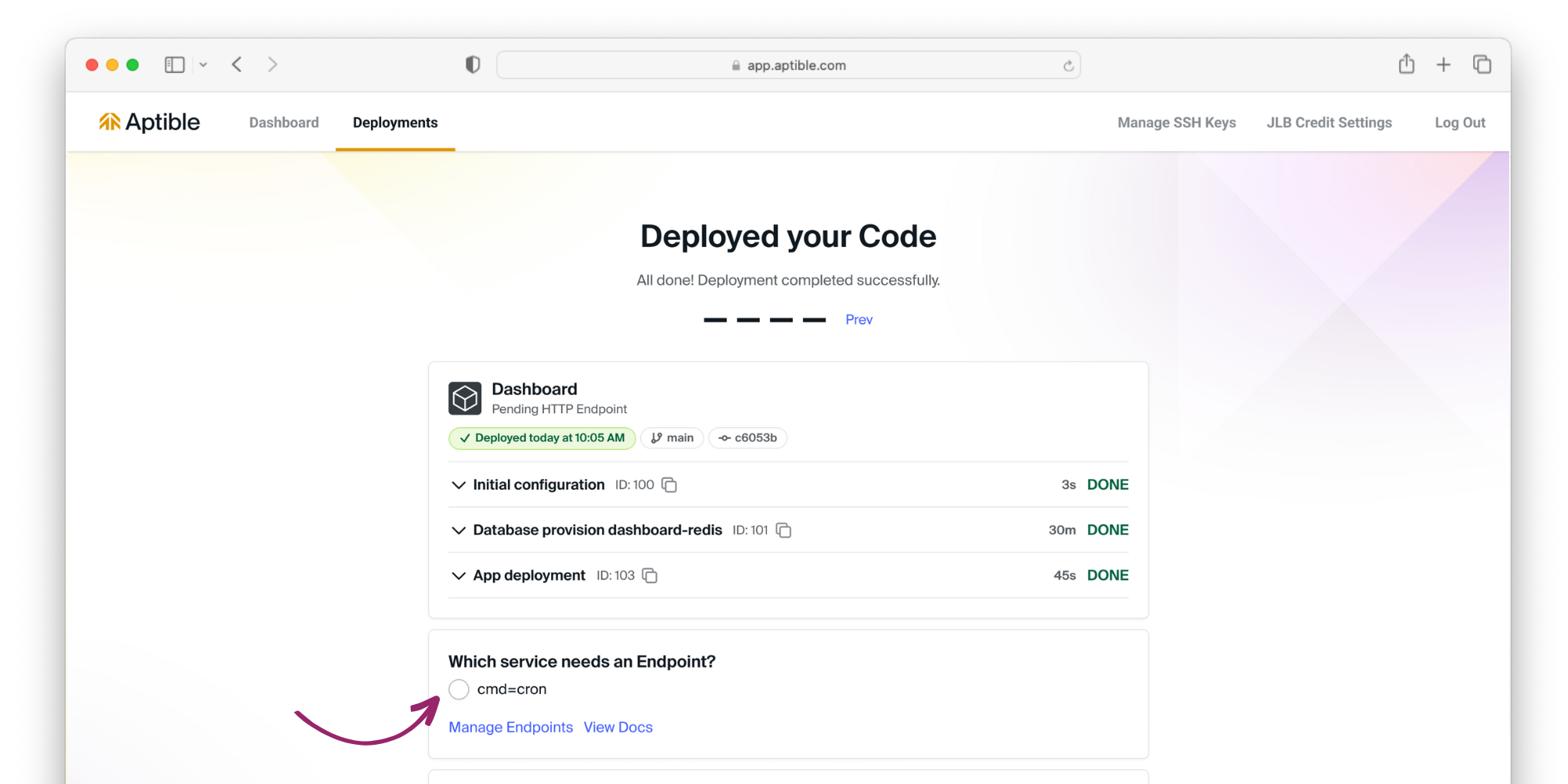
Now that your code is deployed, it's time to expose your app to the internet. Select the service that needs an endpoint, and Aptible will automatically provision a managed endpoint.
Step 08: View your deployed app 🎉
Next steps
Continue your journey on Aptible:
- Deploy your own custom code
- Learn more about how Aptible helps engineering teams
- Explore the Security & Compliance Dashboard
PHP + Laravel - Starter Template
- Overview
- Quick Links
- Deploy Template
- Step 01: Deploy with Git Push
- Step 02: Add an SSH key
- Step 03: Environment Setup
- Step 04: Prepare the template
- Step 05: Fill environment variables and deploy!
- Step 06: View logs in real time
- Step 07: Expose your app to the internet
- Step 08: View your deployed app 🎉
- Next steps